UI Components
ScrollView
UI component for rendering scrollable content (horizontal or vertical).
<ScrollView>
is a UI component for rendering scrollable content. Content can be scrolled vertically
(default) or horizontally
.
Note
A ScrollView can only have a single child element.
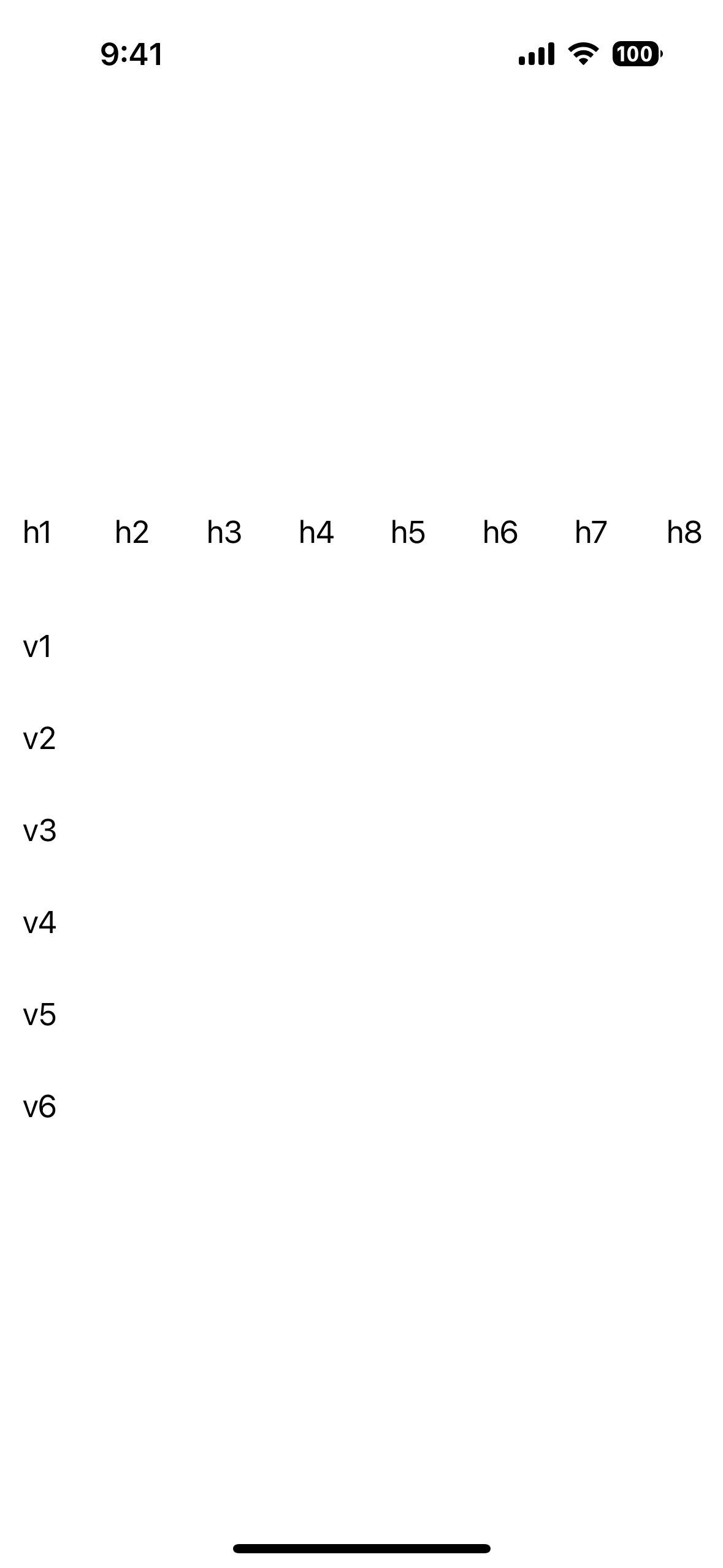
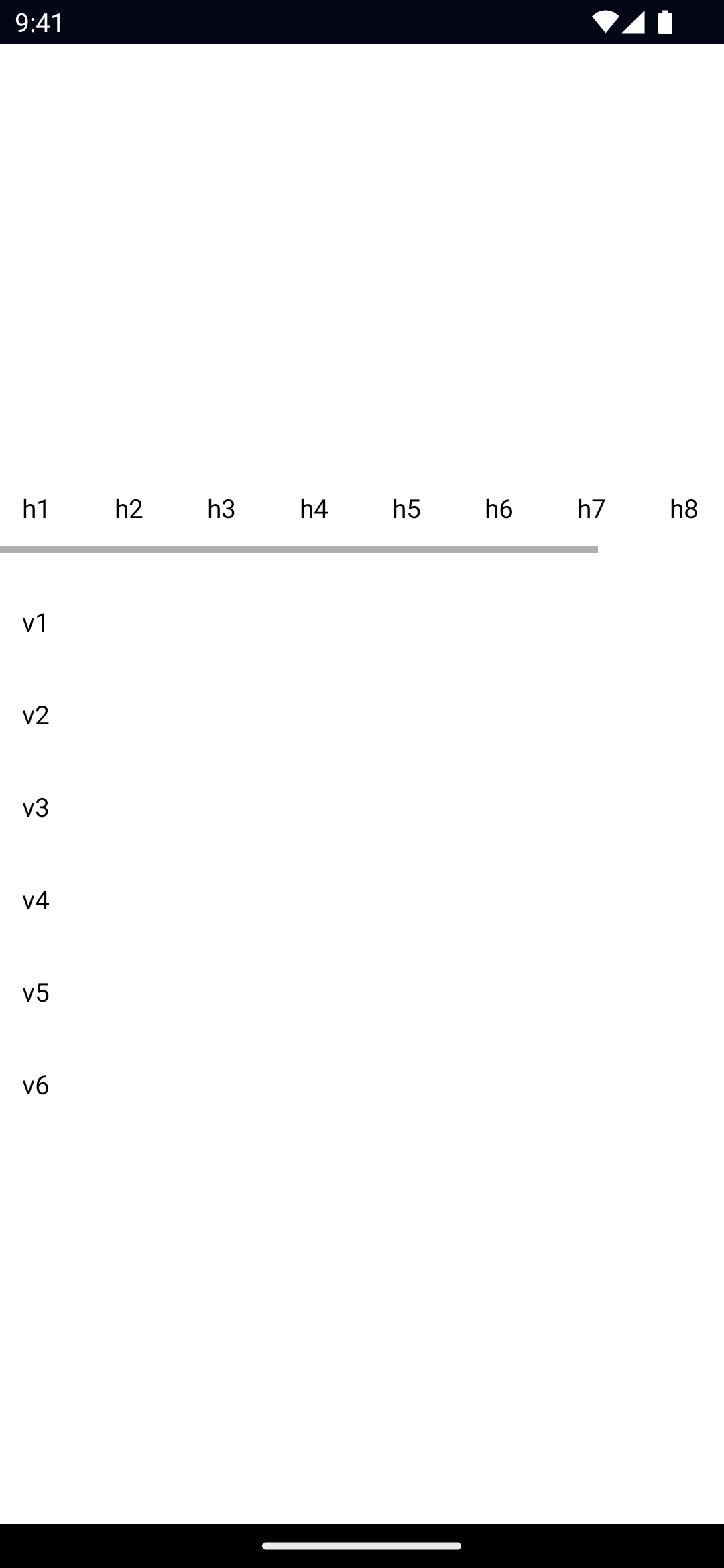
<ScrollView>
<StackLayout padding="12">
<Label text="v1" height="50" />
<Label text="v2" height="50" />
<Label text="v3" height="50" />
<Label text="v4" height="50" />
<Label text="v5" height="50" />
<Label text="v6" height="50" />
<Label text="v7" height="50" />
<Label text="v8" height="50" />
<Label text="v9" height="50" />
</StackLayout>
</ScrollView>
Props
orientation
orientation: 'horizontal' | 'vertical'
Gets or sets the direction in which the content can be scrolled.
Defaults to vertical
.
scrollBarIndicatorVisible
scrollBarIndicatorVisible: boolean
Specifies if the scrollbar is visible.
Defaults to true
.
isScrollEnabled
isScrollEnabled: boolean
Enables or disables scrolling of the ScrollView.
verticalOffset
verticalOffset: number
Gets the vertical offset of the scrolled content.
horizontalOffset
horizontalOffset: number
Gets the horizontal offset of the scrolled content.
scrollableHeight
scrollableHeight: number
Gets the maximum scrollable height, this is also the maximum value for the verticalOffset.
scrollableWidth
scrollableWidth: number = scrollView.scrollableWidth
Gets the maximum scrollable width, this is also the maximum value for the horizontalOffset.
Methods
scrollToVerticalOffset()
scrollToVerticalOffset(value: number, animated: boolean)
Scrolls the content to the specified vertical offset.
Set animated
to true
for animated scroll, false
for immediate scroll.
scrollToHorizontalOffset()
scrollToHorizontalOffset(value: number, animated: boolean)
Scrolls the content to the specified horizontal offset position.
Set animated
to true
for animated scroll, false
for immediate scroll.
Events
scroll
on('scroll', (args: ScrollEventData) => {
const scrollView = args.object as ScrollView
console.log('Scrolled', {
scrollX: args.scrollX,
scrollY: args.scrollY,
})
})
Emitted when the ScrollView is scrolled.
See ScrollEventData.
Native component
- Android:
android.view
- iOS:
UIScrollView